Code from DeepDream.scala:60 executed in 0.00 seconds (0.000 gc):
implicit val _ = log
// First, basic configuration so we publish to our s3 site
log.setArchiveHome(URI.create(s"s3://$s3bucket/${getClass.getSimpleName.stripSuffix("$")}/${log.getId}/"))
log.onComplete(() => upload(log): Unit)
// Fetch input image (user upload prompt)
ImageArtUtil.load(log, contentUrl, resolution)
val canvas = new AtomicReference[Tensor](null)
// Execute the main process while registered with the site index
val registration = registerWithIndexJPG(canvas.get())
try {
// In contrast to other uses, in this painting operation we are enhancing
// an input image (content) only, with no other inputs or canvas preparation.
withMonitoredJpg(() => canvas.get().toImage) {
paint(contentUrl, contentUrl, canvas, new VisualStyleNetwork(
styleLayers = List(
// DeepDream traditionally uses the last layer of a network
VGG16.VGG16_3b
),
styleModifiers = List(
// This operator increases the RMS power of any signal
new ChannelPowerEnhancer()
),
styleUrl = List(contentUrl)
), new BasicOptimizer {
override val trainingMinutes: Int = 60
override val trainingIterations: Int = 20
override val maxRate = 1e9
}, new GeometricSequence {
override val min: Double = resolution
override val max: Double = resolution
override val steps = 1
}.toStream.map(_.round.toDouble): _*)
null
}
} finally {
registration.foreach(_.stop()(s3client, ec2client))
}
}
<function0>
Code from BasicOptimizer.scala:75 executed in 2153.39 seconds (203.437 gc):
val lineSearchInstance: LineSearchStrategy = lineSearchFactory
IterativeTrainer.wrap(trainable)
.setOrientation(orientation())
.setMonitor(new TrainingMonitor() {
override def clear(): Unit = trainingMonitor.clear()
override def log(msg: String): Unit = trainingMonitor.log(msg)
override def onStepFail(currentPoint: Step): Boolean = {
BasicOptimizer.this.onStepFail(trainable, currentPoint)
}
override def onStepComplete(currentPoint: Step): Unit = {
if (0 < logEvery && (0 == currentPoint.iteration % logEvery || currentPoint.iteration < logEvery)) {
val image = currentImage
timelineAnimation += image
val caption = "Iteration " + currentPoint.iteration
out.p(caption + "\n" + out.jpg(image, caption))
}
BasicOptimizer.this.onStepComplete(trainable, currentPoint)
trainingMonitor.onStepComplete(currentPoint)
super.onStepComplete(currentPoint)
}
})
.setTimeout(trainingMinutes, TimeUnit.MINUTES)
.setMaxIterations(trainingIterations)
.setLineSearchFactory((_: CharSequence) => lineSearchInstance)
.setTerminateThreshold(java.lang.Double.NEGATIVE_INFINITY)
.runAndFree
.asInstanceOf[lang.Double]
Reset training subject: 968416920658700
Reset training subject: 968441894640300
Adding measurement 2f10f633 to history. Total: 0
LBFGS Accumulation History: 1 points
Constructing line search parameters: GD+Trust
th(0)=-1.0268817260395504;dx=-2.3745159847053682E-6
New Minimum: -1.0268817260395504 > -1.0268826572015477
WOLFE (weak): th(2.154434690031884)=-1.0268826572015477; dx=-2.3744875988820997E-6 evalInputDelta=9.311619972862673E-7
New Minimum: -1.0268826572015477 > -1.0268853841759686
WOLFE (weak): th(4.308869380063768)=-1.0268853841759686; dx=-2.3745165110185515E-6 evalInputDelta=3.658136418227542E-6
New Minimum: -1.0268853841759686 > -1.0268962255620808
WOLFE (weak): th(12.926608140191302)=-1.0268962255620808; dx=-2.374616718452966E-6 evalInputDelta=1.4499522530408981E-5
New Minimum: -1.0268962255620808 > -1.0269407883148114
WOLFE (weak): th(51.70643256076521)=-1.0269407883148114; dx=-2.3748749234290426E-6 evalInputDelta=5.906227526097929E-5
New Minimum: -1.0269407883148114 > -1.0271777690431394
WOLFE (weak): th(258.53216280382605)=-1.0271777690431394; dx=-2.3738544154182494E-6 evalInputDelta=2.9604300358898605E-4
New Minimum: -1.0271777690431394 > -1.0286627728942133
WOLFE (weak): th(1551.1929768229563)=-1.0286627728942133; dx=-2.3671452451520635E-6 evalInputDelta=0.0017810468546628488
New Minimum: -1.0286627728942133 > -1.03957486080655
WOLFE (weak): th(10858.350837760694)=-1.03957486080655; dx=-2.3877208614547036E-6 evalInputDelta=0.012693134766999625
New Minimum: -1.03957486080655 > -1.1323104148202472
WOLFE (weak): th(86866.80670208555)=-1.1323104148202472; dx=-2.178120190430501E-6 evalInputDelta=0.10542868878069678
New Minimum: -1.1323104148202472 > -1.4744340982187705
END: th(781801.26031877)=-1.4744340982187705; dx=-6.291438147340129E-7 evalInputDelta=0.44755237217922006
Fitness changed from -1.0268817260395504 to -1.4744340982187705
Iteration 1 complete. Error: -1.4744340982187705 Total: 306.2188; Orientation: 0.1535; Line Search: 232.7337
<a id="p-10"></a>Iteration 1
<a id
...skipping 16031 bytes...
jected: LBFGS Orientation magnitude: 7.882e+03, gradient 2.886e-02, dot -0.580; [bf52e911-9681-43ae-836d-a2548dd46213 = 1.000/1.000e+00]
Orientation rejected. Popping history element from -97.15955840449934, -103.60005795169103, -118.70567222979923, -130.42393412691354
LBFGS Accumulation History: 3 points
Removed measurement ac91282 to history. Total: 4
th(0)=-130.42393412691354;dx=-8.326776220487106E-4
Armijo: th(261823.66024693043)=-112.02270022686552; dx=7.461358029576527E-5 evalInputDelta=-18.401233900048013
New Minimum: -130.42393412691354 > -134.6357660733679
WOLF (strong): th(130911.83012346522)=-134.6357660733679; dx=4.7520352421099965E-5 evalInputDelta=4.211831946454367
New Minimum: -134.6357660733679 > -141.13139892428435
END: th(43637.27670782174)=-141.13139892428435; dx=-3.232512311475461E-4 evalInputDelta=10.707464797370818
Fitness changed from -130.42393412691354 to -141.13139892428435
Iteration 19 complete. Error: -141.13139892428435 Total: 119.8871; Orientation: 3.7193; Line Search: 94.0402
Adding measurement 11787b64 to history. Total: 3
Rejected: LBFGS Orientation magnitude: 6.437e+03, gradient 2.450e-02, dot -0.745; [bf52e911-9681-43ae-836d-a2548dd46213 = 1.000/1.000e+00]
Orientation rejected. Popping history element from -103.60005795169103, -118.70567222979923, -130.42393412691354, -141.13139892428435
LBFGS Accumulation History: 3 points
Removed measurement 4998e74b to history. Total: 4
th(0)=-141.13139892428435;dx=-6.001548861486969E-4
New Minimum: -141.13139892428435 > -151.48794654342305
END: th(94013.66271785146)=-151.48794654342305; dx=-2.2008252718138483E-4 evalInputDelta=10.356547619138695
Fitness changed from -141.13139892428435 to -151.48794654342305
Iteration 20 complete. Error: -151.48794654342305 Total: 72.6798; Orientation: 3.7826; Line Search: 46.7637
<a id="p-24"></a>Iteration 20
<a id="p-23"></a>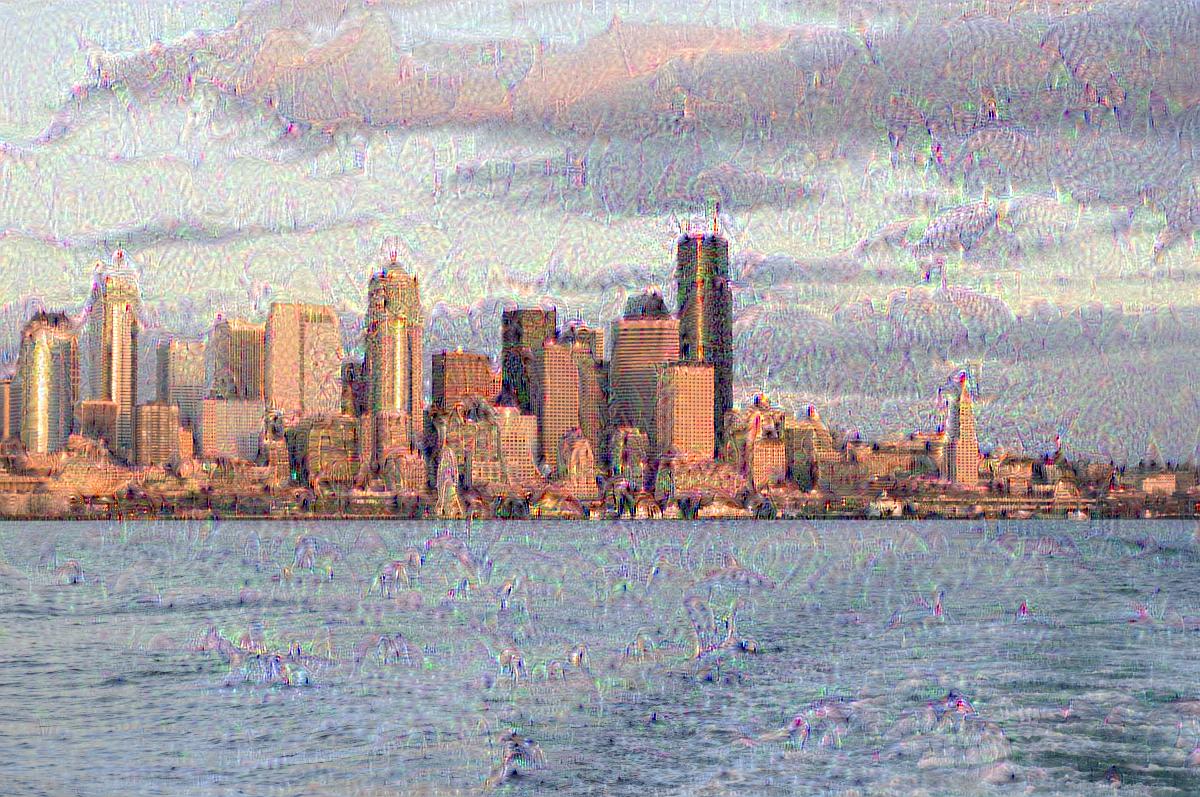
Final threshold in iteration 21: -151.48794654342305 (> -Infinity) after 2153.374s (< 3600.000s)
-151.48794654342305