Code from DeepDream.scala:60 executed in 0.00 seconds (0.000 gc):
implicit val _ = log
// First, basic configuration so we publish to our s3 site
log.setArchiveHome(URI.create(s"s3://$s3bucket/${getClass.getSimpleName.stripSuffix("$")}/${log.getId}/"))
log.onComplete(() => upload(log): Unit)
// Fetch input image (user upload prompt)
ImageArtUtil.load(log, contentUrl, resolution)
val canvas = new AtomicReference[Tensor](null)
// Execute the main process while registered with the site index
val registration = registerWithIndexJPG(canvas.get())
try {
// In contrast to other uses, in this painting operation we are enhancing
// an input image (content) only, with no other inputs or canvas preparation.
withMonitoredJpg(() => canvas.get().toImage) {
paint(contentUrl, contentUrl, canvas, new VisualStyleNetwork(
styleLayers = List(
// DeepDream traditionally uses the last layer of a network
VGG16.VGG16_3b
),
styleModifiers = List(
// This operator increases the RMS power of any signal
new ChannelPowerEnhancer()
),
styleUrl = List(contentUrl)
), new BasicOptimizer {
override val trainingMinutes: Int = 120
override val trainingIterations: Int = 100
override val maxRate = 1e9
}, new GeometricSequence {
override val min: Double = resolution
override val max: Double = resolution
override val steps = 1
}.toStream.map(_.round.toDouble): _*)
null
}
} finally {
registration.foreach(_.stop()(s3client, ec2client))
}
}
<function0>
Code from BasicOptimizer.scala:75 executed in 7226.49 seconds (629.387 gc):
val lineSearchInstance: LineSearchStrategy = lineSearchFactory
IterativeTrainer.wrap(trainable)
.setOrientation(orientation())
.setMonitor(new TrainingMonitor() {
override def clear(): Unit = trainingMonitor.clear()
override def log(msg: String): Unit = trainingMonitor.log(msg)
override def onStepFail(currentPoint: Step): Boolean = {
BasicOptimizer.this.onStepFail(trainable, currentPoint)
}
override def onStepComplete(currentPoint: Step): Unit = {
if (0 < logEvery && (0 == currentPoint.iteration % logEvery || currentPoint.iteration < logEvery)) {
val image = currentImage
timelineAnimation += image
val caption = "Iteration " + currentPoint.iteration
out.p(caption + "\n" + out.jpg(image, caption))
}
BasicOptimizer.this.onStepComplete(trainable, currentPoint)
trainingMonitor.onStepComplete(currentPoint)
super.onStepComplete(currentPoint)
}
})
.setTimeout(trainingMinutes, TimeUnit.MINUTES)
.setMaxIterations(trainingIterations)
.setLineSearchFactory((_: CharSequence) => lineSearchInstance)
.setTerminateThreshold(java.lang.Double.NEGATIVE_INFINITY)
.runAndFree
.asInstanceOf[lang.Double]
Reset training subject: 970856626177600
Reset training subject: 970883175103400
Adding measurement 441fbe89 to history. Total: 0
LBFGS Accumulation History: 1 points
Constructing line search parameters: GD+Trust
th(0)=-1.0268817260395504;dx=-2.3745159847053682E-6
New Minimum: -1.0268817260395504 > -1.0268826572015477
WOLFE (weak): th(2.154434690031884)=-1.0268826572015477; dx=-2.3744875988820997E-6 evalInputDelta=9.311619972862673E-7
New Minimum: -1.0268826572015477 > -1.0268853841759686
WOLFE (weak): th(4.308869380063768)=-1.0268853841759686; dx=-2.3745165110185515E-6 evalInputDelta=3.658136418227542E-6
New Minimum: -1.0268853841759686 > -1.0268962255620808
WOLFE (weak): th(12.926608140191302)=-1.0268962255620808; dx=-2.374616718452966E-6 evalInputDelta=1.4499522530408981E-5
New Minimum: -1.0268962255620808 > -1.0269407883148114
WOLFE (weak): th(51.70643256076521)=-1.0269407883148114; dx=-2.3748749234290426E-6 evalInputDelta=5.906227526097929E-5
New Minimum: -1.0269407883148114 > -1.0271777690431394
WOLFE (weak): th(258.53216280382605)=-1.0271777690431394; dx=-2.3738544154182494E-6 evalInputDelta=2.9604300358898605E-4
New Minimum: -1.0271777690431394 > -1.0286627728942133
WOLFE (weak): th(1551.1929768229563)=-1.0286627728942133; dx=-2.3671452451520635E-6 evalInputDelta=0.0017810468546628488
New Minimum: -1.0286627728942133 > -1.03957486080655
WOLFE (weak): th(10858.350837760694)=-1.03957486080655; dx=-2.3877208614547036E-6 evalInputDelta=0.012693134766999625
New Minimum: -1.03957486080655 > -1.1323104148202472
WOLFE (weak): th(86866.80670208555)=-1.1323104148202472; dx=-2.178120190430501E-6 evalInputDelta=0.10542868878069678
New Minimum: -1.1323104148202472 > -1.4744340982187705
END: th(781801.26031877)=-1.4744340982187705; dx=-6.291438147340129E-7 evalInputDelta=0.44755237217922006
Fitness changed from -1.0268817260395504 to -1.4744340982187705
Iteration 1 complete. Error: -1.4744340982187705 Total: 303.8460; Orientation: 0.1546; Line Search: 228.2295
<a id="p-10"></a>Iteration 1
<a id
...skipping 67463 bytes...
magnitude: 7.173e+03, gradient 7.231e-02, dot -0.668; [0f5d81a6-3a2f-43cd-aca0-d3f02e2fa404 = 1.000/1.000e+00]
Orientation rejected. Popping history element from -675.0386854520332, -678.3813505706349, -697.7843913343651, -710.0871207372411
LBFGS Accumulation History: 3 points
Removed measurement 7893c715 to history. Total: 4
th(0)=-710.0871207372411;dx=-0.005229409916387462
Armijo: th(50222.231340893784)=-679.0977770337367; dx=5.947093191775028E-4 evalInputDelta=-30.989343703504346
New Minimum: -710.0871207372411 > -712.2067051044044
END: th(25111.115670446892)=-712.2067051044044; dx=-4.115521522324228E-4 evalInputDelta=2.11958436716327
Fitness changed from -710.0871207372411 to -712.2067051044044
Iteration 74 complete. Error: -712.2067051044044 Total: 93.9200; Orientation: 3.6842; Line Search: 67.4977
Adding measurement 467af68c to history. Total: 3
Rejected: LBFGS Orientation magnitude: 6.344e+03, gradient 8.836e-02, dot -0.561; [0f5d81a6-3a2f-43cd-aca0-d3f02e2fa404 = 1.000/1.000e+00]
Orientation rejected. Popping history element from -678.3813505706349, -697.7843913343651, -710.0871207372411, -712.2067051044044
LBFGS Accumulation History: 3 points
Removed measurement 274bae2c to history. Total: 4
th(0)=-712.2067051044044;dx=-0.007807106566526661
Armijo: th(54100.25870581403)=-628.7564053453656; dx=0.0031041573007599943 evalInputDelta=-83.45029975903878
Armijo: th(27050.129352907014)=-710.3858417627345; dx=0.001936742445806811 evalInputDelta=-1.8208633416699058
New Minimum: -712.2067051044044 > -730.2359424469703
END: th(9016.709784302338)=-730.2359424469703; dx=-0.0029060642817826127 evalInputDelta=18.02923734256592
Fitness changed from -712.2067051044044 to -730.2359424469703
Iteration 75 complete. Error: -730.2359424469703 Total: 114.4869; Orientation: 3.6810; Line Search: 88.9366
<a id="p-46"></a>Iteration 75
<a id="p-45"></a>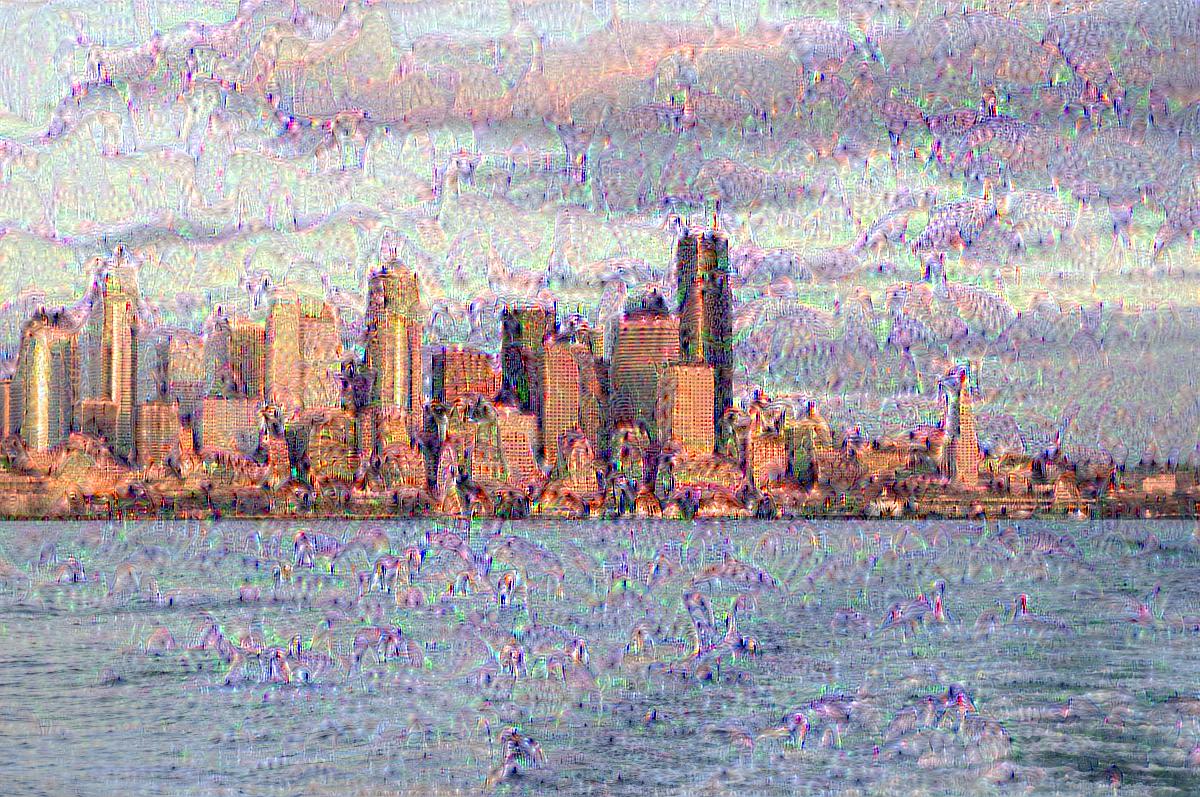
Final threshold in iteration 75: -730.2359424469703 (> -Infinity) after 7226.478s (< 7200.000s)
-730.2359424469703